Now it differs from addition...
"double" vector sorting with class defined function is faster than inline function defined in header file. See the below test.
Test:Copy and paste the below codes to your
previous test project files to add vector sorting capability.
İf you want, change the name of the class "Add" in "MyMath.h". Since it contains also sort() function now
Do not forget to include "vector" and "algortihm" for dealing with vector and its sort() method.
Here is the additional includes for changed files
#include"vector"
#include "algorithm"
//put this code to "MyMath.h" int sort_vector_inline(vector<double>& v){ //defined inline sort(v.begin(),v.end()); return 1; }
int sort_vector_class_def(vector<double>& v);
===============================================
//put this code to "class_def.cpp" int sort_vector_class_def(vector<double>& v){ //defined inline sort(v.begin(),v.end()); return 1; }
===============================================
//All of Main.cpp is given here.(lots of changes). //Filename:Main.cpp
#include "MyMath.h"
#include "stdafx.h"
#include "vector"
#include "algorithm"
using namespace std;
void main()
{
DWORD startTime,endTime,difTime;
vector v,v1;
int a=1;
int b=2;
int c;
Add testAdd;
cout<<"double vector sorting..."<<"\n";
//fill vector with random numbers
for(int j=0;j<1e7;j++){
v1.push_back(rand());
}
startTime=GetTickCount();
testAdd.sort_vector_inline(v1);
endTime=GetTickCount();
difTime=endTime-startTime;
cout<< "it took "<//fill vector again since sorted vector sorting is much more faster
for(int j=0;j<1e7;j++){
v.push_back(rand());
}
startTime=GetTickCount();
testAdd.sort_vector_class_def(v);
endTime=GetTickCount();
difTime=endTime-startTime;
cout<< "it took "<
cout<<"addition..."<<"\n";
//use function defined in other cpp file
startTime=GetTickCount();
for(int i=0;i<1e9;i++){
c=testAdd.add_class_def(a,b);
}
endTime=GetTickCount();
difTime=endTime-startTime;
cout<< "it took "<startTime=GetTickCount();
for(int i=0;i<1e9;i++){ style="color: rgb(0, 0, 0);"> c=testAdd.add_inline(a,b);
}
endTime=GetTickCount();
difTime=endTime-startTime;
cout<< "it took "<}
===============================================
I changed the order of functions in main file to see if there is a difference in speed.
It is same for the addition. Still inline function works faster.
Let's see what happened for vector sorting (below pictures).
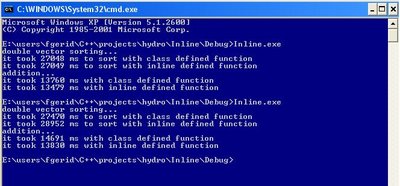

if you change the order of function calling sequence, inline function for vector sorting works faster.
So we could not say anything about speeds yet.
Labels: C, C++, Programming